前言
栈是一种基于 FILO(先进后出)的数据结构,是一种只能在一端进行插入和删除操作的特殊线性表。它按照先进后出的原则存储数据,先进入的数据被压入栈底,最后的数据在栈顶,需要读数据的时候从栈顶开始弹出数据(最后一个数据被第一个读出来)。
一、栈
我们称数据进入到栈的动作称为压栈,数据从栈中出去的动作为弹栈,如下图:
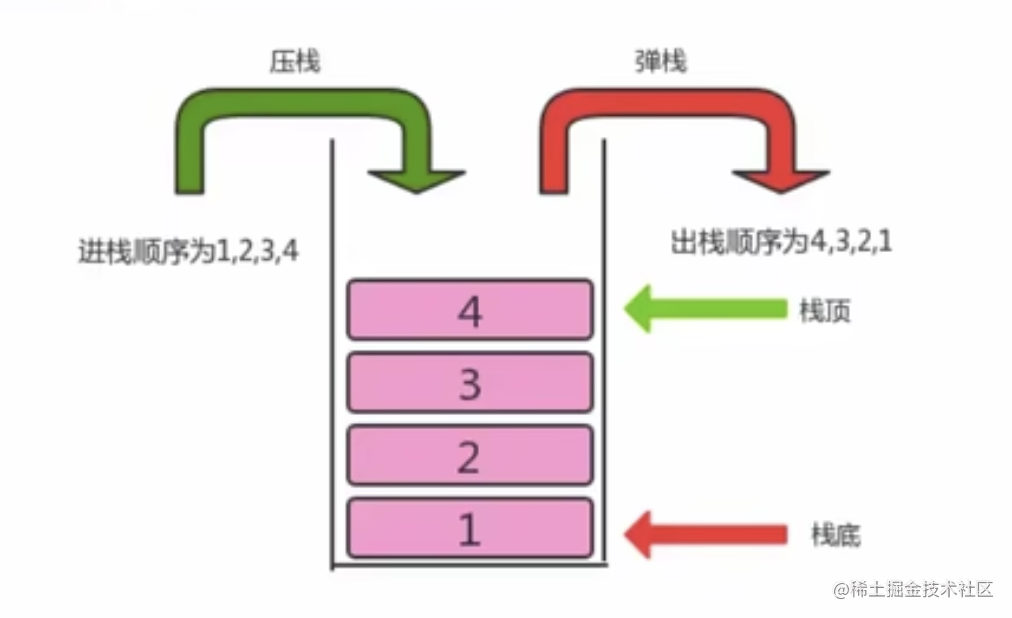
1.1、栈 API 设计
类名 |
Stack<T> |
构造方法 |
Stack() :创建 Stack 对象 |
成员方法 |
1、public boolean isEmpty() :判断栈是否为空 2、public int size() :获取栈中元素的个数 3、public T pop() :弹出栈顶元素 4、public void push(T t) :向栈中压入元素 t |
成员变量 |
1、private Node head :记录头节点 2、private int N :当前栈的元素个数 |
成员内部类 |
private class Node :节点类 |
1.2、栈代码实现
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52
| public class Stack<T>{
private Node head; private int N;
public Stack() { head = new Node(null, null); N = 0; }
private class Node{ public Node next; public T item;
public Node(Node next, T item) { this.next = next; this.item = item; } }
public boolean isEmpty(){ return N == 0; }
public int size(){ return N; }
public void clear(){ head.next = null; N = 0; }
public void push(T item){ Node temp = head.next; Node newNode = new Node(null, item); head.next = newNode; newNode.next = temp; N++; }
public T pop(){ Node temp = head.next; if(temp == null)return null; head.next = temp.next; N--; return temp.item; } }
|
1.3、栈遍历
我们也让 Stack 支持增强 for 循环:
1、让 Stack 实现 Iterable 接口,重写 iterator 接口
2、在 Stack 内部提供一个内部类 SIterator,实现 Iterator 接口,重写 hasNext() 方法和 next() 方法
具体实现:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79
| public class Stack<T> implements Iterable<T>{
private Node head; private int N;
public Stack() { head = new Node(null, null); N = 0; }
private class Node{ public Node next; public T item;
public Node(Node next, T item) { this.next = next; this.item = item; } }
public boolean isEmpty(){ return N == 0; }
public int size(){ return N; }
public void clear(){ head.next = null; N = 0; }
public void push(T item){ Node temp = head.next; Node newNode = new Node(null, item); head.next = newNode; newNode.next = temp; N++; }
public T pop(){ Node temp = head.next; if(temp == null)return null; head.next = temp.next; N--; return temp.item; }
@NonNull @Override public Iterator<T> iterator() { return new SIterator(); }
private class SIterator implements Iterator<T>{
private Node temp;
public SIterator() { temp = head; }
@Override public boolean hasNext() { return temp.next != null; }
@Override public T next() { temp = temp.next; return temp.item; } } }
|
1.4、栈测试
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28
| public class StackTest {
public static void main(String[] args) { Stack<String> integerStack = new Stack<>(); integerStack.push("a"); integerStack.push("b"); integerStack.push("c"); integerStack.push("d"); for (String str : integerStack) { System.out.println(str); }
System.out.println(integerStack.pop()); System.out.println(integerStack.pop());
integerStack.clear(); System.out.println(integerStack.size()); } }
|
二、总结
本篇文章我们介绍了:
1、栈:一种先进后出的数据结构
2、栈的 API 设计,代码实现,增强 for 循环,测试
好了,本篇文章到这里就结束了,感谢你的阅读🤝